数组
声明
1 2 3
| String[] aArray = new String[5]; String[] bArray = {"a","b","c", "d", "e"}; String[] cArray = new String[]{"a","b","c","d","e"};
|
根据数组创建ArrayList
1 2 3 4
| String[] stringArray = { "a", "b", "c", "d", "e" }; List<String> arrayList = new ArrayList<String>(Arrays.asList(stringArray)); System.out.println(arrayList);
|
数组内是否包含某个值
1 2 3
| String[] stringArray = { "a", "b", "c", "d", "e" }; boolean b = Arrays.asList(stringArray).contains("a"); System.out.println(b);
|
注意:
1 2 3 4
| int[] int_arr = {1,2,3,4}; Integer[] integer_arr = {1,2,3,4}; System.out.println(Arrays.asList(int_arr).contains(1)); System.out.println(Arrays.asList(integer_arr).contains(1));
|
声明
声明
声明
声明
ArrayList
ArrayList 类是一个可以动态修改的数组,与普通数组的区别就是它是没有固定大小的限制,我们可以添加或删除元素。
ArrayList 继承了 AbstractList ,并实现了 List 接口。
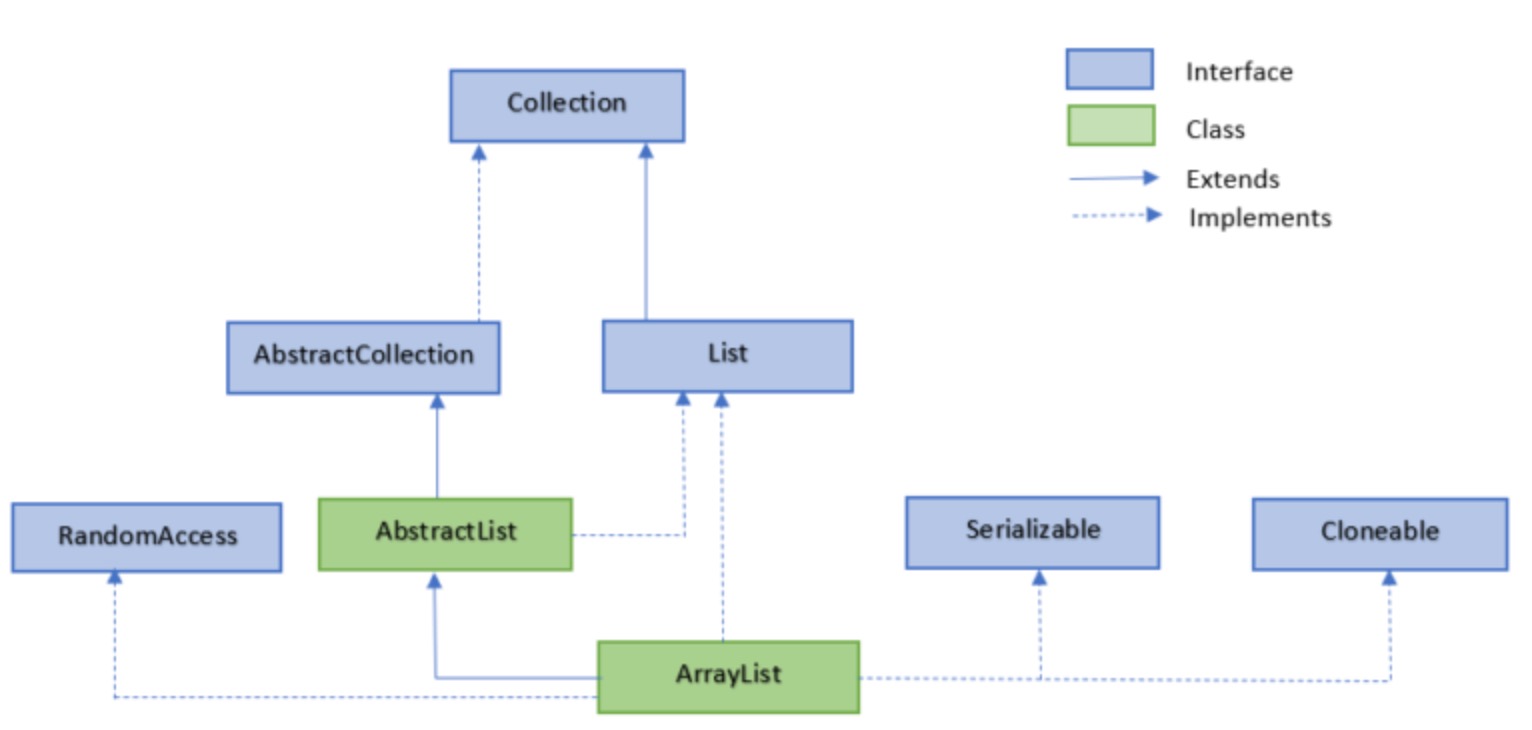
常用操作
- 添加元素:
list.add(index, element)
,(index不传入的话默认添加到List的最后)
- 删除元素:
list.remove(index)
,删除指定位置的元素,或者使用list.removeAll()
删除所有元素
- 修改元素:
list.set(index, element)
,替换指定位置的元素
- 获取元素:
list.get(index)
- 获取size:
list.size()
- 遍历:
for(Type element : lists)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| List<Integer> list01 = new ArrayList<Integer>(); list01.add(1); list01.add(2); list01.add(3); list01.add(4); list01.add(5); System.out.println(list01); System.out.println(list01.get(2));
list01.add(2, 6); System.out.println(list01);
list01.remove(3); System.out.println(list01);
list01.set(0, 10); System.out.println(list01); System.out.println(list01.size());
|
其他常用操作
方法 |
描述 |
addAll() |
添加集合中的所有元素到 arraylist 中 |
clear() |
删除 arraylist 中的所有元素 |
clone() |
复制一份 arraylist |
contains() |
判断元素是否在 arraylist |
indexOf() |
返回 arraylist 中元素的索引值 |
isEmpty() |
判断 arraylist 是否为空 |
subList() |
截取部分 arraylist 的元素 |
sort() |
对 arraylist 元素进行排序 |
toArray() |
将 arraylist 转换为数组 |
toString() |
将 arraylist 转换为字符串 |
ensureCapacity() |
设置指定容量大小的 arraylist |
lastIndexOf() |
返回指定元素在 arraylist 中最后一次出现的位置 |
retainAll() |
保留 arraylist 中在指定集合中也存在的那些元素 |
containsAll() |
查看 arraylist 是否包含指定集合中的所有元素 |
trimToSize() |
将 arraylist 中的容量调整为数组中的元素个数 |
removeRange() |
删除 arraylist 中指定索引之间存在的元素 |
replaceAll() |
将给定的操作内容替换掉数组中每一个元素 |
removeIf() |
删除所有满足特定条件的 arraylist 元素 |
forEach() |
遍历 arraylist 中每一个元素并执行特定操作 |
Map
创建
1
| Map<Integer, Integer> numMap = new HashMap<>();
|
添加元素
判断是否包含key
1 2 3
| if (numMap.containsKey(key)){ }
|